There are at least five ways to make AJAX calls with the jQuery library. For beginners, however, the differences between each can be a bit confusing. In this tutorial, we’ll line them up and make a comparison. Additionally. we’ll review how to inspect these AJAX calls with Firebug as well.
What is AJAX
This section is for those who have no idea what AJAX is. If you don’t fall into this category, feel free to skip to the next section.
AJAX stands for asynchronous JavaScript and XML. If you see another term XHR, which is shorthand for XML HTTP request, it’s the same thing. Don’t be afraid of this jargon; AJAX is not rocket science.
- In Gmail, switch from inbox to draft. Part of the page is changed, but the page is not refreshed. You remain on the same page. Url has not changed (except for the #draft at the end of the url, but that’s still the same webpage).
- In Google Reader, select a feed. The content changes, but you are not redirected to another url.
- In Google Maps, zoom in or zoom out. The map has changed, but you remain on the same page.
The key to AJAX’s concept is “asynchronous”. This means something happens to the page after it’s loaded. Traditionally, when a page is loaded, the content remains the same until the user leaves the page. With AJAX, JavaScript grabs new content from the server and makes changes to the current page. This all happena within the lifetime of the page, no refresh or redirection is needed.
Caching AJAX
Now we should know what AJAX actually is. And we know that, when Gmail refreshes some content without redirection, an AJAX call is made behind the scenes. The requested content can either be static (remains exactly the same all the time, such as a contact form or a picture) or dynamic (requests to the same url get different responses, such as Gmail’s inbox where new mails may show up any time).
For static content, we may want the response cached. But for dynamic content, which can change in a second’s time, caching AJAX becomes a bug, right? It should be noted that Internet Explorer always caches AJAX calls, while other browsers behave differently. So we’d better tell the browser explicitly whether or not AJAX should be cached. With jQuery, we can accomplish this simply by typing:
- $.ajaxSetup ({
- cache: false
- });
1. load(): Load HTML From a Remote URL and Inject it into the DOM
The most common use of AJAX is for loading HTML from a remote location and injecting it into the DOM. With jQuery’s load() function, this task is a piece of cake. Review this demo and we’ll go over some uses one by one.
Minimal Configuration
Click on the first button named “load().” A piece of HTML is injected into the page, exactly what we were talking about. Let’s see what’s going on behind the scenes.
Below is the JavaScript code for this effect:
- $.ajaxSetup ({
- cache: false
- });
- var ajax_load = "
";
-
- // load() functions
- var loadUrl = "ajax/load.php";
- $("#load_basic").click(function(){
- $("#result").html(ajax_load).load(loadUrl);
- });
- $.ajaxSetup forces the browser NOT to cache AJAX calls.
- After the button is clicked, it takes a little while before the new HTML is loaded. During the loading time, it’s best to show an animation to provide the user with some feedback to ensure that the page is currently loading. The “ajax_load” variable contains the HTML of the loading sign.
- “ajax/load.php” is the url from which the HTML is grabbed.
- When the button is clicked, it makes an AJAX call to the url, receives the response HTML, and injects it into the DOM. The syntax is simply $(“#DOM”).load(url). Can’t be more straightforward, hah?
Now, let’s explore more details of the request with Firebug:
- Open Firebug.
- Switch to the “Net” tab. Enable it if it’s disabled. This is where all HTTP request in the browser window are displayed.
- Switch to “XHR” tab below “Net”. Remember the term “XHR?” It’s the request generated from an AJAX call. All requests are displayed here.
- Click on the “load()” button and you should see the following.
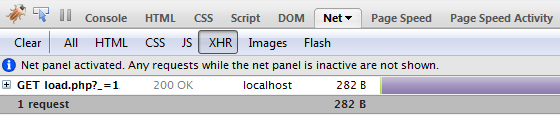
The request is displayed, right? Click on the little plus sign to the left of the request, more information is displayed.
Click on the “Params” tab. Here’s all parameters passed through the GET method. See the long number string passed under a “_” key? This is how jQuery makes sure the request is not cached. Every request has a different “_” parameter, so browsers consider each of them to be unique.
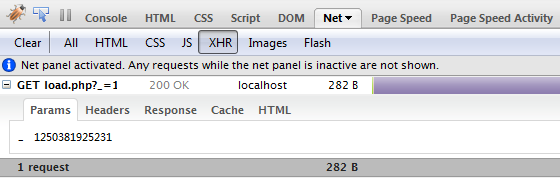
Click on the “Response” tab. Here’s the HTML response returned from the remote url.
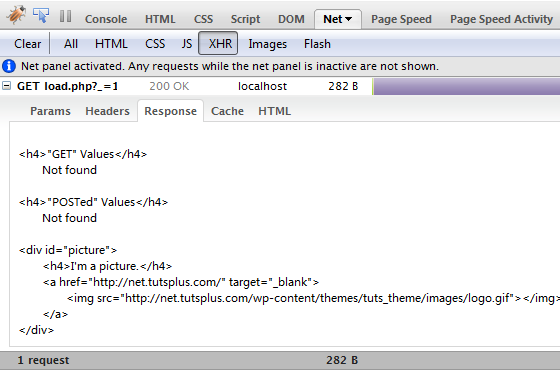
Load Part of the Remote File
Click on “load() #DOM” button. We notice that only the Envato link is loaded this time. This is done with the following code:
- $("#load_dom").click(function(){
- $("#result")
- .html(ajax_load)
- .load(loadUrl + " #picture");
- });
With load(url + “#DOM”), only the contents within #DOM are injected into current page.
Pass Parameters Through the GET Method
Click on the “load() GET” button and open firebug.
- $("#load_get").click(function(){
- $("#result")
- .html(ajax_load)
- .load(loadUrl, "language=php&version=5");
- });
By passing a string as the second param of load(), these parameters are passed to the remote url in the GET method. In Firebug, these params are shown as follows:
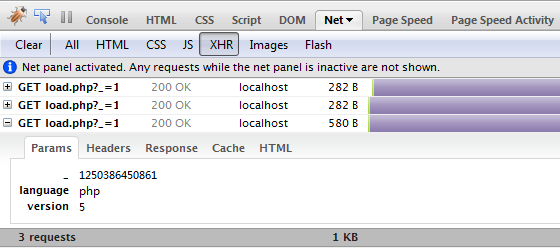
Pass Parameters Through the POST Method
Click on the “load() POST” button and open Firebug.
- $("#load_post").click(function(){
- $("#result")
- .html(ajax_load)
- .load(loadUrl, {language: "php", version: 5});
- });
If parameters are passed as an object (rather than string), they are passed to the remote url in the POST method.
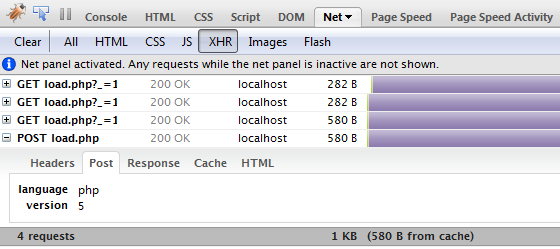
Do Something on AJAX Success
Click on “load() callback” button.
- $("#load_callback").click(function(){
- $("#result")
- .html(ajax_load)
- .load(loadUrl, null, function(responseText){
- alert("Response:\n" + responseText);
- });
- });
A function can be passed to load() as a callback. This function will be executed as soon as the AJAX request is completed successfully.
2. $.getJSON(): Retrieve JSON from a Remote Location
Now we’ll review the second AJAX method in jQuery.
JSON (JavaScript Object Notation) is a lightweight data-interchange format. It’s very convenient when exchanging data programmatically with JSON.
Let’s review an example.
Find the $.getJSON() section in the demo page, type in some words in your native language, and click detect language.
- // $.getJSON()
- var jsonUrl = "ajax/json.php";
- $("#getJSONForm").submit(function(){
- var q = $("#q").val();
- if (q.length == 0) {
- $("#q").focus();
- } else {
- $("#result").html(ajax_load);
- $.getJSON(
- jsonUrl,
- {q: q},
- function(json) {
- var result = "Language code is \"" + json.responseData.language + "\"";
- $("#result").html(result);
- }
- );
- }
- return false;
- });
Let’s jump to Line 9:
- $.getJSON doesn’t load information directly to the DOM. So the function is $.getJSON, NOT $(“#result”).getJSON. (There are pairs of similar looking functions in jQuery such as $.each() and each(). Check out their respective documentation for more information.)
- $.getJSON accepts three parameters. A url, parameters passed to the url and a callback function.
- $.getJSON passes parameters in GET method. POSTing is not possible with $.getJSON.
- $.getJSON treats response as JSON.
$.getJSON’s function name is NOT camel-cased. All four letters of “JSON” are in uppercase.
Look at the response in JSON format in Firebug. It’s returned from Google Translate API. Check out ajax/json.php in source files to see how language detection works.
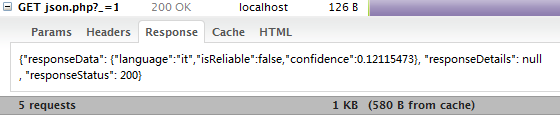
3. $.getScript(): Load JavaScript from a Remote Location
We can load JavaScript files with $.getScript method. Click on “Load a Remote Script” button in the demo page; let’s review the code for this action.
- // $.getScript()
- var scriptUrl = "ajax/script.php";
- $("#getScript").click(function(){
- $("#result").html(ajax_load);
- $.getScript(scriptUrl, function(){
- $("#result").html("");
- });
- });
- $.getScript accepts only two parameters, a url, and a callback function.
- Neither the GET nor POST params can be passed to $.getScript. (Of course you can append GET params to the url.)
- JavaScript files don’t have to contain the “.js” extension. In this case, the remote url points to a PHP file. Let your imagination fly and you can dynamically generate JavaScript files with PHP.
See the response JavaScript in Firebug.

4. $.get(): Make GET Requests
$.get() is a more general-purpose way to make GET requests. It handles the response of many formats including xml, html, text, script, json, and jonsp. Click on the “$.get()” button in the demo page and see the code.
- // $.get()
- $("#get").click(function(){
- $("#result").html(ajax_load);
- $.get(
- loadUrl,
- {language: "php", version: 5},
- function(responseText){
- $("#result").html(responseText);
- },
- "html"
- );
- });
- $.get() is completely different, as compared to get(). The latter has nothing to do with AJAX at all.
- $.get accepts the response type as the last parameter, which makes it more powerful than the first functions we introduced today. Specify response type if it’s not html/text. Possible values are xml, html, text, script, json and jonsp.
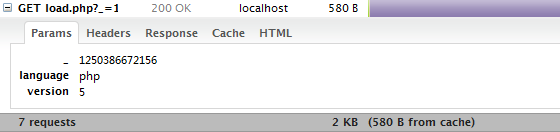
5. $.post(): Make POST Requests
$.post() is a more general-purpose way to make POST requests. It does exactly the same job as $.get(), except for the fact that it makes a POST request instead.
- // $.post()
- $("#post").click(function(){
- $("#result").html(ajax_load);
- $.post(
- loadUrl,
- {language: "php", version: 5},
- function(responseText){
- $("#result").html(responseText);
- },
- "html"
- );
- });
The use of $.post() is the same as its brother, $.get(). Check the POST request in Firebug (shown in the following image).
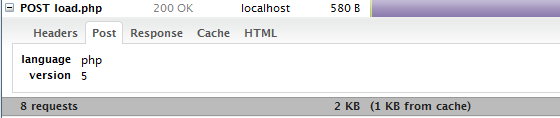
Finally… $.ajax():
Up to this point, we’ve examined five commonly used jQuery AJAX functions. They bear different names but, behind the scenes, they generally do the exact same job with slightly different configurations. If you need maximum control over your requests, check out the $.ajax() function.
This is jQuery’s low-level AJAX implementation. See $.get, $.post etc. for higher-level abstractions that are often easier to understand and use, but don’t offer as much functionality (such as error callbacks). -jQuery’s official Documentation
In my opinion, the first five functions should satisfy most of our needs. But if you need to execute a function on AJAX error, $.ajax() is your only choice.
Conclusion
Today, we took an in-depth look of five ways to make AJAX calls with jQuery.
- load(): Load a piece of html into a container DOM.
- $.getJSON(): Load a JSON with GET method.
- $.getScript(): Load a JavaScript.
- $.get(): Use this if you want to make a GET call and play extensively with the response.
- $.post(): Use this if you want to make a POST call and don’t want to load the response to some container DOM.
- $.ajax(): Use this if you need to do something when XHR fails, or you need to specify ajax options (e.g. cache: true) on the fly.
Before we conclude, here’s a comparison table of these functions. I hope you enjoyed this lesson! Any thoughts?
No comments:
Post a Comment