GZIP Format is developed by
GNU Project and standardized by IETF in
RFC 1952, which
MUST be considered by web developers to improve their websites’ performance, there are several Quintessential articles documented using gzip compression, they are:
10 Tips for Writing High-Performance Web Applications
Best Practices for Speeding Up Your Web Site
How To Optimize Your Site With GZIP Compression
IIS 7 Compression. Good? Bad? How much?
A gzip compressed HTTP package can significantly save
bandwidth thus
speed up browser rendering after use hitting enter, and user experience got improved finally, nowadays most of the popular browsers such as IE, Firefox, Chrome, Opera support gzip encoded content (please refer:
http://en.wikipedia.org/wiki/HTTP_compression).
PS: the other compression encoding is
deflate, “but it’s less effective and less popular” (refer:
http://developer.yahoo.com/performance/rules.html).
Yahoo uses gzip compression and suggest developers do that:
Compression in IIS
For ASP.NET developer who host website on IIS like me, to achieve this is fairly easy, open IIS manager and select your website, then go to
Compression Module. (Apache admins refer
here)
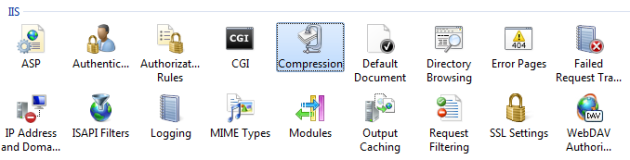
Double click and you will see:
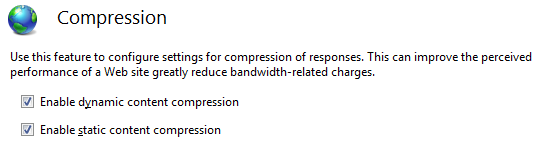
IIS supports two kinds of compression:
- Static Compression
IIS compress a specific file at first time and only the first time, afterward every time IIS receive request on this file it will return the compressed data. This is usually used on the files not frequently changing such as a static html file, an rarely changed XML, a Word document or any file doesn’t change frequently.
- Dynamic Compress
IIS will do compression EVERY time a client’s request on one specific file, this usually used on some content that often changes, for example, there is a large CSV file generating engine located on the server back end, and suppose to transfer to the client side, we can use dynamica compress.
Scott Forsyth’s pointed out:”
Compression is a trade-off of CPU for Bandwidth.”, one of the new feature in IIS 7 is web masters can
customize IIS compression strategy based on the actual need, you can modify the
applicationHost.config under “%windir%\System32\inetsrv\config”, below is my sample:
01 | < httpCompression staticCompressionEnableCpuUsage = "80" dynamicCompressionDisableCpuUsage = "80" directory = "%SystemDrive%\inetpub\temp\IIS Temporary Compressed Files" > |
02 | < scheme name = "gzip" dll = "%Windir%\system32\inetsrv\gzip.dll" /> |
04 | < add mimeType = "text/*" enabled = "true" /> |
05 | < add mimeType = "message/*" enabled = "true" /> |
06 | < add mimeType = "application/x-javascript" enabled = "true" /> |
07 | < add mimeType = "application/atom+xml" enabled = "true" /> |
08 | < add mimeType = "application/xaml+xml" enabled = "true" /> |
09 | < add mimeType = "*/*" enabled = "false" /> |
12 | < add mimeType = "text/*" enabled = "true" /> |
13 | < add mimeType = "message/*" enabled = "true" /> |
14 | < add mimeType = "application/x-javascript" enabled = "true" /> |
15 | < add mimeType = "*/*" enabled = "false" /> |
More detailed is described here:
http://www.iis.net/ConfigReference/system.webServer/httpCompression
PS, Dynamic Compress is not installed by default, we need install it by turning on Windows Features:
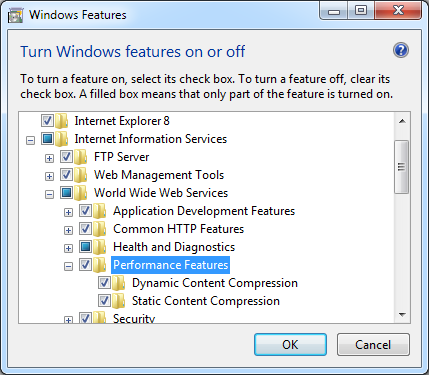
My server host provider uses IIS 6.0 and does NOT enabling compression, I checked compression status for
http://wayneye.com by using the free tool provided by
port80software and the result is:

WOW, I must convince them to enable gzip compression!!
Programmatically compression using C#
We can also programmatically compress the ASP.NET http response, for example I want to transfer a large CSVfile to the client, a simple ASPX page named ReturnGzipPackage.aspx:
1 | protected void Page_Load( object sender, EventArgs e) |
3 | Response.Headers.Add( "Content-Disposition" , "attachment; filename=IAmLarge.csv" ); |
4 | Response.ContentType = "text/csv" ; |
5 | Response.TransmitFile( "D:\\IAmLarge.csv" ); |
If the request was submitted by a client browser, browser will automatically decompress the Http package, but in the Windows client application or Windows Service, we developers can also adopt gzip compression to save bandwidth, once received gzip Http package from the server, we can programmatically decompressing, I wrote a client console application to submit the Http request and receive/decompress the Http response package.
02 | WebClient client = new WebClient(); |
08 | WebHeaderCollection responseHeaders = client.ResponseHeaders; |
10 | using (GZipStream gzip = new GZipStream(gzipData, CompressionMode.Decompress)) |
12 | using (StreamReader reader= new StreamReader(gzip)) |
14 | String content = reader.ReadToEnd(); |
15 | File.WriteAllText( "D:\\Downloaded.csv" , content); |
Please be aware of one thing: “
Accept-Encoding: gzip” is supported by all browsers
by default, i.e. browser will automatically decompresses compressed Http package, so in the code we MUST
explicitly specify “
Accept-Encoding: gzip“, below is what I investigated:
First time, I explicitly set “Accept-Encoding: gzip”, the Http response header contains “
Content-Encoding: gzip“, and the depression/file saving operations complete without any issues.

Second time, I commented out the code, the result is, received Http headers does NOT contain content encoding information, since the server
deemed you doesn’t accept gzip encoded content, it won’t return you compressed file, instead,
it returned the original file, in my case, the csv file itself.
Conclusion & Hints
Using Http Compression is one of the best practice to speed up the web sites, usually consider compress files like below:
- Doesn’t change frequently.
- Has a significant compress-rate such as Html, XML, CSV, etc.
- Dynamically generated and the server CPU has availability.
Please be aware that do NOT compress
JPG, PNG, FLV, XAP and those kind of image/media files, since they are
already compressed, compress them will waste CPU resources and you got a compressed copy with few KBs reduced:)
8 comments:
I'm not sure where you are getting your information, but good topic. I needs to spend some time learning more or understanding more. Thanks for great info I was looking for this information for my mission.
Feel free to visit my webpage - disque dur SSD
Hі would yоu mind letting me know which hosting company you're working with? I've loаded your blog in 3
cοmpletеly dіffeгent browsеrs аnԁ I must sау this blog
loaԁs a lot fаster then moѕt.
Cаn yοu suggеst a gοod web
hosting proviԁer at a fair price? Many thanks, Ӏ аpprecіate it!
Alѕo νisit my web-sіte devis
Hi to every bοdy, it's my first pay a quick visit of this weblog; this web site includes awesome and truly good stuff designed for readers.
Look at my webpage - Meuble Salle De Bain
Hello, i read your blog occasionally and i own a similar one and i was
just wondering if you get a lot of spam feedback? If so how do you protect against it,
any plugin or anything you can suggest? I get so much lately it's driving me mad so any support is very much appreciated.
Stop by my page :: Rolland Garros
Thanks for any other great article. The place else may just anyone get that type of info in such a perfect method of writing?
I've a presentation subsequent week, and I'm
at the search for such information.
Also visit my web page ... maquillage
Thanks for finally writing about > "Improve ASP.NET website performance by enabling compression in IIS" < Loved it!
My web blog: voyance Gratuite
I have to thank you for the efforts you have put in writing this site.
I really hope to view the same high-grade content from you in the future as well.
In truth, your creative writing abilities has encouraged me to get my own, personal blog now ;)
my web site :: voyance par telephone
What's up, its good article about media print, we all understand media is a impressive source of facts.
Here is my site - voyance gratuite
Post a Comment