jTemplate is a template engine plug-in for jQuery and is commonly used to display tabular data by binding objects to the template. Before using jTemplate, you must know how to use the jTemplate syntax. The following tags are supported in jTemplate and you can see an example here
· if..elseif..else../if
· foreach..else../for
· for..else../for
· continue, break
· include
· paramcycle
· derim .. rdelim
· literal
The latest version of jQuery script can be downloaded over here. Visual Studio 2010 comes with jQuery 1.4.1 and I will be using this version for this article. Assuming you have to downloaded jTemplate Plug-in, let’s start.
Step 1: Open Visual Studio 2010. Create new ASP.NET 4 Web site from File > New > WebSite. First, I have created a Patient class which will return the Patient List as shown below.
C#
using System;
using System.Collections.Generic;
Public Class Patients
{
public List getList()
{
List Patients = new List();
Patients.Add(new Patient { ID = 1, FullName = "Aamir Hasan", Address = "-", PhoneNo = "3335494532", Gender = 'M' });
Patients.Add(new Patient { ID = 2, FullName = "Awais Ahmed", Address = "-", PhoneNo = "-", Gender = 'M' });
Patients.Add(new Patient { ID = 3, FullName = "Amir Hassan", Address = "-", PhoneNo = "12345678", Gender = 'M' });
Patients.Add(new Patient { ID = 4, FullName = "Sobia Hina", Address = "-", PhoneNo = "-", Gender = 'F' });
Patients.Add(new Patient { ID = 5, FullName = "Mahwish Hasan", Address = "-", PhoneNo = "65789544", Gender = 'F' });
Patients.Add(new Patient { ID = 6, FullName = "Saba Khan", Address = "-", PhoneNo = "12345678", Gender = 'F' });
Patients.Add(new Patient { ID = 7, FullName = "John ", Address = "-", PhoneNo = "877467889", Gender = 'M' });
Patients.Add(new Patient { ID = 8, FullName = "Salman Khan", Address = "-", PhoneNo = "44772798", Gender = 'M' });
Patients.Add(new Patient { ID = 9, FullName = "Nasir Hameed", Address = "-", PhoneNo = "543287", Gender = 'M' });
return Patients;
}
}
Public Class Patient
{
public int ID { get; set; }
public String FullName { get; set; }
public string Address { get; set; }
public string PhoneNo { get; set; }
public char Gender { get; set; }
}
VB.NET
Imports System
Imports System.Collections.Generic
Public ReadOnly Property Patients() As Class
public List(Of Patient) getList()
Dim Patients_Renamed As New List(Of Patient)()
Patients_Renamed.Add(New Patient With {.ID = 1, .FullName = "Aamir Hasan", .Address = "-", .PhoneNo = "3335494532", .Gender = "M"c})
Patients_Renamed.Add(New Patient With {.ID = 2, .FullName = "Awais Ahmed", .Address = "-", .PhoneNo = "-", .Gender = "M"c})
Patients_Renamed.Add(New Patient With {.ID = 3, .FullName = "Amir Hassan", .Address = "-", .PhoneNo = "12345678", .Gender = "M"c})
Patients_Renamed.Add(New Patient With {.ID = 4, .FullName = "Sobia Hina", .Address = "-", .PhoneNo = "-", .Gender = "F"c})
Patients_Renamed.Add(New Patient With {.ID = 5, .FullName = "Mahwish Hasan", .Address = "-", .PhoneNo = "65789544", .Gender = "F"c})
Patients_Renamed.Add(New Patient With {.ID = 6, .FullName = "Saba Khan", .Address = "-", .PhoneNo = "12345678", .Gender = "F"c})
Patients_Renamed.Add(New Patient With {.ID = 7, .FullName = "John ", .Address = "-", .PhoneNo = "877467889", .Gender = "M"c})
Patients_Renamed.Add(New Patient With {.ID = 8, .FullName = "Salman Khan", .Address = "-", .PhoneNo = "44772798", .Gender = "M"c})
Patients_Renamed.Add(New Patient With {.ID = 9, .FullName = "Nasir Hameed", .Address = "-", .PhoneNo = "543287", .Gender = "M"c})
Return Patients_Renamed
End Property
Public Property Patient() As Class
public Integer ID {get;set;}
public String FullName {get;set;}
public String Address {get;set;}
public String PhoneNo {get;set;}
public Char Gender {get;set;}
End Property
Step 2: Open your Default.aspx.cs/vb page and create a static function which will return a patient list to the jQuery function from the server-side to client-side.
C#
using System.Web.Services;
[WebMethod]
public static List getAllPatientList()
{
Patients pObj = new Patients();
return pObj.getList();
}
VB.NET
Imports System.Web.Services
Public Shared Function getAllPatientList() As List(Of Patient)
Dim pObj As New Patients()
Return pObj.getList()
End Function
Note: Add a reference to the System.Web.Services in the codebehind of Default.aspx, to allow calls from client script.
Here’s a preview of the collection returned when you run the application.
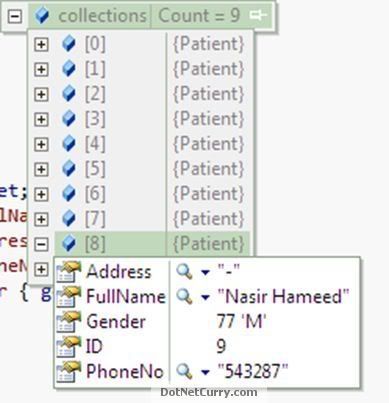
Step 3: Now create a ‘ForEachTemplate.htm’ page in which we will add a table template to be processed by jTemplate, and the data will be populated and displayed on the browser. I have also applied CSS on this table.
<table border="0" cellpadding="0" cellspacing="0" id="table1">
<thead>
<tr>
<th>Idth>
<th>Full Nameth>
<th>Addressth>
<th>PhoneNoth>
<th>Genderth>
tr>
thead>
<tbody>
{#foreach $T as Patient}
<tr bgcolor="{#cycle values=['#fff','#f7f7f7']}">
<td>
{ $T.Patient.ID }
td>
<td>
{ $T.Patient.FullName }
td>
<td>
{ $T.Patient.Address }
td>
<td>
{ $T.Patient.PhoneNo }
td>
<td>
{ $T.Patient.Gender }
td>
tr>
{#/for}
tbody>
table>
Now open your Default.aspx Page and add the jQuery and jTemplate reference as shown below:
<asp:Content ID="HeaderContent" runat="server" ContentPlaceHolderID="HeadContent">
<script src="Scripts/jquery-1.4.1.js" type="text/javascript">script>
<script src="Scripts/jtemplates.js" type="text/javascript">script>
<script type="text/javascript">
$(document).ready(function () {
$.ajax({
type: "POST",
url: "Default.aspx/getAllPatientList",
data: "{}",
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (data) {
$('#placeholder').setTemplateURL('JTemplates/ForEachTemplate.htm');
$('#placeholder').processTemplate(data.d);
}
});
});
script>
asp:Content>
The function accepts the JSON results retrieved from the Web Service and uses a jTemplate method to load the HTML template into a container, and finally renders the JSON result. To display the results, add a div element called ‘placeholder’ as shown below:
<asp:Content ID="BodyContent" runat="server" ContentPlaceHolderID="MainContent">
<h2>jTemplate,jQuery and JSON in ASP.NET h2>
<p>
<div id="placeholder" style="clear: both;">div>
p>
asp:Content>
No comments:
Post a Comment