Message Queuing is a message infrastructure and a development platform for creating distributed messaging applications for the Microsoft Windows Operating System. Message Queuing applications can use the Message Queuing infrastructure to communicate heterogeneous networks and with computers that may be offline. Message Queuing provides guaranteed message delivery, efficient routing, security, transaction support and priority based messaging. Administrative Privileges are required to create a queue.
With the release of MS Windows 2000, the name of Microsoft's message queuing solution changed from Microsoft Messaging Queue Server to Message Queuing. In the Windows Platform SDK, the term Messaging Queuing refers to all versions of the product. There are three different types of Message Queuing versions:
- MSMQ 1.0: Microsoft Message Queue Server 1.0 product operates with MS Windows NT 4.0, Microsoft MS Windows 95, MS Windows 98, and MS Windows Me.
- MSMQ 2.0: the Message Queuing service included in MS Windows 2000.
- MSMQ 3.0: Message queuing service included in MS Windows XP Professional and Windows Server 2003 family.
When to use Message Queue?
Message Queuing is useful when the client application is often disconnected from the network. For example, salespersons can enter order data directory at the customer's site. The application sends a message for each order to the message queue that is located on the client's system.
Message Queuing can also be useful in a connected environment. For example, the server of a website is fully loaded with order transactions at some specific time periods, say evening times or morning times, but the load is low at night time. The solution to this problem would be to buy a faster server or add an additional server to the system. However, the cheaper solution would be sending message queue.
There are different types of Message Queues:
- Normal Message
- Acknowledgement Message
- Respond Message
- Report Message
A message can have a priority that defines the order in which the message will be read from a queue. Commonly, messages are stored on the disk can be found in the \system32\msmq\stored directory.
Message Queuing Features
Message Queuing is a part of the Windows Operating System. Main features of this service are:
- Messages can be sent in a disconnected environment. It is not necessary for the sending and receiving application to run at the same time.
- A program can assign different priorities to messages when it puts the message in the queue.
- Using Express mode, messages can be sent very fast. Express mode messages are just stored in memory.
- Message Queues can be secured with access control lists to define which users can send or receive messages from a queue. We can also encrypt the data to avoid network sniffers from reading the data.
- We can send the messages using guaranteed delivery. Recoverable messages are stored within files. They are delivered even in case the server reboots.
- Message Queuing 3.0 supports sending multicast messages.
Message Queuing Products Message Queuing 3.0 is a part of Windows Server 2003 and Windows XP, Windows 2000 comes with the Message Queuing 2.0, which did not have support for the HTTP protocol and multicast messages. You can install a message queue in Windows XP using Add or Remove Programs, a separate section within windows components where Message Queuing options can be selected. Within the message Queuing options, various components can be selected (refer Fig 1 and Fig 2).
Active Directory Integration:
Within this feature, message queue names are written to the Active Directory integration and to secure queues with Windows users and groups
Common:
The common subcomponent is required for base functionality with Message Queuing
MSMQHTTP Support:
This support allows you to send and receive messages using the HTTP protocol.
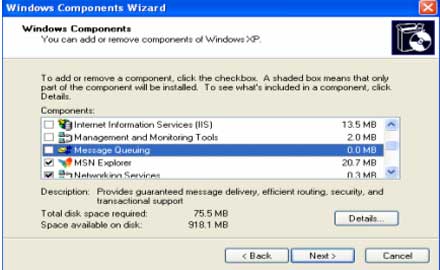
Figure 1
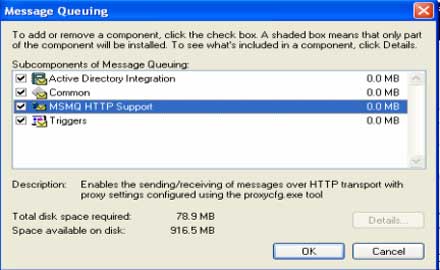
Figure 2
Triggers:
With Triggers applications can be instantiated on the arrival of a new message.
When Message Queuing is installed, the Message Queuing Service must be started. This service reads and writes messages and communicates with other Message Queuing servers to route messages across the network.
Now we will see how to send and receiving messages using C# programming.
Programming Message Queuing
Creating a Message Queue
In C#, we can also create Message Queues programmatically using the Create() method of MessageQueue class. With Create() method, the path of the new queue must be passed. The path consists of the hostname where the queue is located and the name of the queue. Now we will write an example, which will create on the localhost that is 'MynewPublicQueue'. To create a private queue, the path name must include private$. For example: \private$\MynewPrivateQueue.
Programming Message Queuing
Creating a Message Queue
In C#, we can also create Message Queues programmatically using the Create() method of MessageQueue class. With Create() method, the path of the new queue must be passed. The path consists of the hostname where the queue is located and the name of the queue. Now we will write an example, which will create on the localhost that is 'MynewPublicQueue'. To create a private queue, the path name must include private$. For example: \private$\MynewPrivateQueue.
Once the create() method is invoked, properties of the queue can be changed. Using label property, the label of the queue is set to "First Queue". The following program writes the path of the queue and the format name to the console. The format name is automatically created with a UUID (Universal Unique Identifiers) that can be used to access the queue without the name of the server. Check out Code 1.
Code 1:
Code 1:
using System;
using System.Collections.Generic;
using System.Messaging;
using System.Text;
namespace FirstQueue
{
class Program
{
static void Main(string[] args)
{
using (MessageQueue queue = MessageQueue.Create(@". \myqueue"))
{
queue.Label = "First Queue";
Console.WriteLine("Queue Created:");
Console.WriteLine("Path: {0}, queue.Path");
Console.Writeline("FormatName: {0}, queue.FormatName");
}
}
}
}
Finding a Queue
To identify the queue, you can use the path name and format name. You can also differentiate between public and private queues. Public queues are published in the Active directory. For these queues, it is not necessary to know the system where they are located.
Private queues can be found only in the name of the system where the queues located are known. You can find the public queues in the Active directory by searching for the queue's table, category or format name. You can also get all the queues on the machine.
The class MessageQueue has static methods to search for queues:
To identify the queue, you can use the path name and format name. You can also differentiate between public and private queues. Public queues are published in the Active directory. For these queues, it is not necessary to know the system where they are located.
Private queues can be found only in the name of the system where the queues located are known. You can find the public queues in the Active directory by searching for the queue's table, category or format name. You can also get all the queues on the machine.
The class MessageQueue has static methods to search for queues:
- GetPublicQueuesByLable()
- GetPublicQueuesByCategory()
- GetPublicQueuesByMachine()
The method GetPublicQueues() return an array of all public queues in the domain.
Code 2:
Code 2:
using System;
using System.Messaging;
namespace FirstQueue
{
class Program
{
static void Main(string[] args)
{
foreach (MessageQueue queue in MessageQueue.GetPublicQueues())
{
Console.WriteLine("queue.Path");
}
}
}
}
You can also make queues private using the static method called GetPrivateQueuesByMachine(). This method returns all private queues from a specific system.
Opening Known Queues
If you the name of the queue, then it is not necessary to search for it. Queues can be opened using the path or format name.
Pathname
Pathname specifies the machine name and queue name to open the queue. The following code 3 opens the queue FirstQueue on local host. To make sure the queue exists, you use the static method MessageQueue.Exists().
Code 3:
Pathname specifies the machine name and queue name to open the queue. The following code 3 opens the queue FirstQueue on local host. To make sure the queue exists, you use the static method MessageQueue.Exists().
Code 3:
using System;
using System.Messaging;
namespace FirstQueue
{
class Program
{
static void Main(string[] args)
{
if (MessageQueue.Exists(@".\\FirstQueue"))
{
MessageQueue queue = new MessageQueue(@".\\FirstQueue");
}
else
{
Console.WriteLine("Queue .\\FirstQueue not Found");
}
}
}
}
We can use different type identifiers when the queues are opened. Table 1 shows the syntax of the queue name for specific types.
Format Name
Format name can be given to open queue. The formatname is used for searching the queue in the Active Directory to get the host where the queue is located. In a disconnected environment, where the queue cannot be reached at the time the message is sent, it is necessary to use the format name.
Syntax of the format name queue is:
MessageQueue queue= new MessageQueue( @ "FormatName : Public=023423DFG-0984-3w45-K987-12638NU979HH")
Table 1 | |
Queue Type | Syntax |
Private queue | MachineName\Private$\QueueName |
Public queue | MachineName\QueueName |
Journal queue | MachineName\QueueName\Journal$ |
Machine Journal queue | MachineName\Journal$ |
Machine dead letter queue | MachineName\DeadLetter$ |
Machine transactional dead-letter queue | MachineName\XactDeadLetter$ |
Sending a Message
By using the Send method of the MessageQueue class, you can send the message to the queue. The object passed as an argument of the Send() Method is serialized queue. The Send() method is overloaded so that a Label and a MessageQueueTransaction object can be passed. Now we will write a small example to check if the queue exists and if it doesn't exist, a queue is created. Then the queue is opened and the message "First Message" is sent to the queue using the Send() method.
The path name specifies "." for the server name, which is the local system. Path name to private queues only works locally. Add the following code 4 in your Visual Studio C# console environment.
Code 4:
using System;
using System.Messaging;
namespace FirstQueue
{
class Program
{
static void Main(string[] args)
{
try
{
if (!MessageQueue.Exists(@".\Private$\FirstQueue"))
{
MessageQueue.Create(@".\Private$\FirstQueue");
}
MessageQueue queue = new MessageQueue(@".\Private$FirstQueue");
queue.Send("First Message ", " Label ");
}
catch (MessageQueueException ex)
{
Console.WriteLine(ex.Message);
}
}
}
}
Build the application and view and run. You can view the message in the Component Management admin tool as shown in figure 3. By opening the message and selecting the body tab of the dialog, you can see the message was formatted using XML.
Receiving Messages
MessageQueue class can be used for reading messages. With the Receive() method, a single message is read and removed from the queue. We will write a small example to read the Private queue FirstQueue.
When you read a message using the XmlMessageFormatter, we have to pass the types of the objects that are read to the constructor or the formatter. In this example (Code 5), the type System.String is passed to the argument array if the XmlMessageFormatter constructor. The following program is read with the Receive() method and then the message body is written to the console.
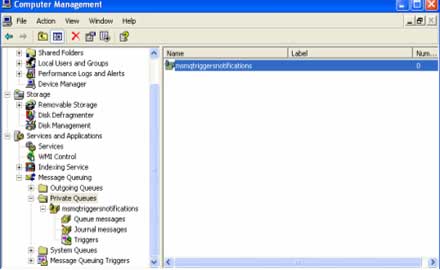
Figure 3
Code 5:
Code 5:
using System;
using System.Messaging;
namespace FirstQueue
{
class Program
{
static void Main(string[] args)
{
MessageQueue queue = new MessageQueue (@".\Private$\FirstQueue");
Queue.Formatter = new XmlMessageFormatter( new string[]("System.String"));
Message Mymessage = queue.Receive();
Console.WriteLine(Mymessage.Body);
}
}
}
Receive() message behaves synchronously and waits until a message is in the queue if there is none. Try out the rest in Visual Studio 2005.
Conclusion
Message Queues can be created with MessageQueue.Create() method. But to create a Message queue you should have administrative rights.
No comments:
Post a Comment